The BFF service serves an unexisting user interface, yet we can imagine what it needs to do; it must:
- Serve the product catalog so customers can browse the shop.
- Serve a specific product to render a product details page.
- Serve the list of items in a user’s shopping cart.
- Enable users to manage their shopping cart by adding, updating, and removing items.
Of course, the list of features could go on, like allowing the users to purchase the items, which is the ultimate goal of an e-commerce website. However, we are not going that far. Let’s start with the catalog.
Fetching the catalog
The catalog acts as a routing gateway and forwards the requests to the Products downstream service.The first endpoint serves the whole catalog by using our typed client (highlighted):
app.MapGet(
“api/catalog”,
(IWebClient client, CancellationToken cancellationToken)
=> client.Catalog.FetchProductsAsync(cancellationToken)
);
Sending the following requests should hit the endpoint:
GET https://localhost:7254/api/catalog
The endpoint should respond with something like the following:
HTTP/1.1 200 OK
Content-Type: application/json; charset=utf-8
{
“products”: [
{
“id”: 2,
“name”: “Apple”,
“unitPrice”: 0.79
},
{
“id”: 1,
“name”: “Banana”,
“unitPrice”: 0.30
},
{
“id”: 3,
“name”: “Habanero Pepper”,
“unitPrice”: 0.99
}
]
}
Here’s a visual representation of what happens:
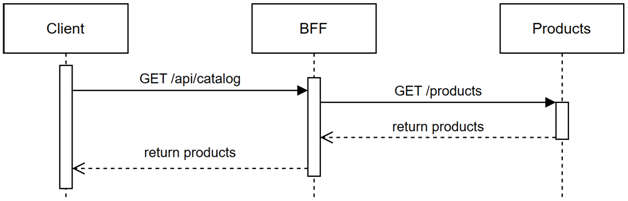
Figure 19.27: a sequence diagram representing the BFF routing the request to the Products service
The other catalog endpoint is very similar and also simply routes the request to the correct downstream service:
app.MapGet(
“api/catalog/{productId}”,
(int productId, IWebClient client, CancellationToken cancellationToken)
=> client.Catalog.FetchProductAsync(new(productId), cancellationToken)
);
Sending an HTTP call will result in the same as calling it directly because the BFF only acts as a router.We explore more exciting features next.